We are going to build an Angular Application with all the CRUD Operations, that is Create, Read, Update, Delete.
We are going to build this Angular Form application with Python as the back-end and MySql as the database
If you are interested in knowing about how to create back-end API using python & flask check this link http://datacyper.com/index.php/2020/03/15/create-a-crud-based-app-using-flask-python-mysql/
Now that we have built our API, we can build our other part of the application, to make this a full-stack application.
Before we jump in the code of Angular Form, you have to be careful about ‘@angular/forms’ as you may find yourself debugging errors related to forms.
Also, we are going to use the Axios package so please install it `npm install Axios
We are also going to use some bootstrap components so make sure to add bootstrap links in your index.html file
Add ReactiveFormsModule into your app.module.ts
import { FormsModule, ReactiveFormsModule, FormControl } from '@angular/forms';
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
ReactiveFormsModule
],
Here is the app.component.html code
<h1>Welcome to Angular + Flask + MySql App</h1>
<div class="container">
<form name="registerForm" [formGroup]="registerForm" >
<div class="form-group">
<label for="name">First Name</label>
<input type="text" class="form-control" id="ffirstname" formControlName="ffirstName" [(ngModel)]="ffirstName">
</div>
<div class="form-group">
<label for="alterEgo">Last Name</label>
<input type="text" class="form-control" id="llastname" formControlName="llastName" [(ngModel)]="llastName">
</div>
<button type="submit" class="btn btn-success" (click)="Post()">Submit</button>
<button type="submit" class="btn btn-warning" (click)="Update()">Update</button>
<button type="submit" class="btn btn-danger" (click)="Delete()">Delete</button>
<button type="submit" class="btn btn-primary" (click)="SearchById()">Search By Id</button>
<div class="form-group">
<label for="alterEgo">Search By Id</label>
<input type="text" class="form-control" id="iid" formControlName="iid" [(ngModel)]="iid">
</div>
</form>
<br />
<br />
<button type="submit" class="btn btn-success" (click)="GetAll()">Get All</button>
<table border="1px;" class="table table-hover">
<thead>
<tr>
<td>ID</td>
<td>First Name</td>
<td>Last Name</td>
</tr>
</thead>
<tbody>
<tr *ngFor = "let hero of getall">
<td>{{hero.NameID}}</td>
<td>{{hero.firstname}}</td>
<td>{{hero.lastname}}</td>
</tr>
</tbody>
</table>
</div>
Here is the typescript code of app.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, Validators, FormControl } from '@angular/forms';
import axios from 'axios';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
title = 'angular-flask';
registerForm: FormGroup;
getall: object[];
ffirstName: string;
llastName: string;
iid: string;
xx:string;
constructor(
private formBuilder: FormBuilder
// public NameId: number,
// public FirstName: string,
// public LastName: string,
) {}
ngOnInit() {
this.registerForm = this.formBuilder.group({
ffirstName: ['', [Validators.required]],
llastName: ['', [Validators.required]],
iid: ['', [Validators.required]]
//byname: ['', [Validators.required]],
});
}
GetAll() {
//alert("Hello World");
axios.get('http://localhost:5000/select')
.then( (response)=> {
console.log(response.data);
this.getall = Object.values(response.data);
})
.catch( (error)=> {
console.log(error);
})
}
Post() {
axios.post('http://localhost:5000/insert', {
firstname: this.ffirstName,
lastname: this.llastName
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
alert(error);
console.log(error);
});
}
Update() {
axios.put('http://localhost:5000/update/'+this.iid, {
firstname: this.ffirstName,
lastname: this.llastName
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
alert(error);
console.log(error);
});
}
SearchById() {
axios.get('http://localhost:5000/select/'+this.iid)
.then( (response) => {
console.log(response.data[0].firstname);
this.ffirstName = response.data[0].firstname;
this.llastName = response.data[0].lastname;
})
.catch(function (error) {
alert(error);
console.log(error);
});
}
}
We will get our Application look as below
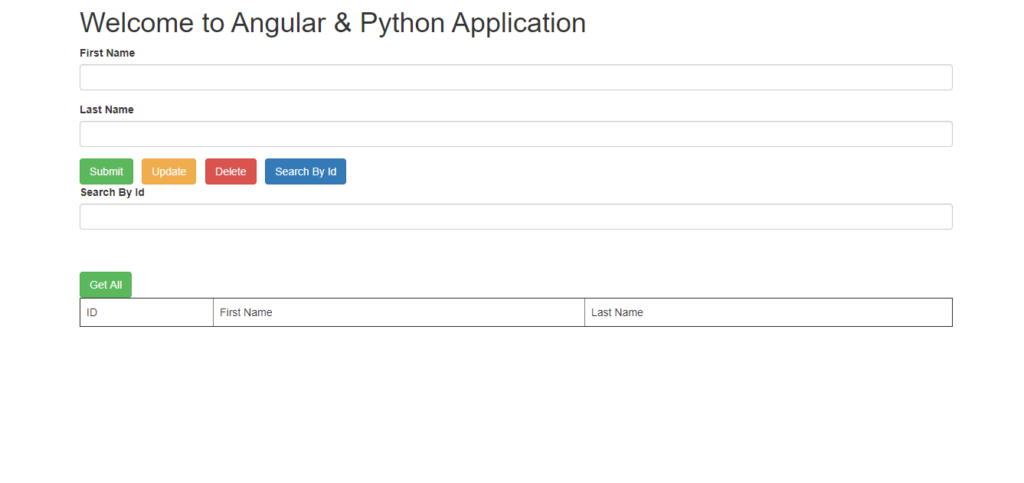
Our Application is now functional, check below
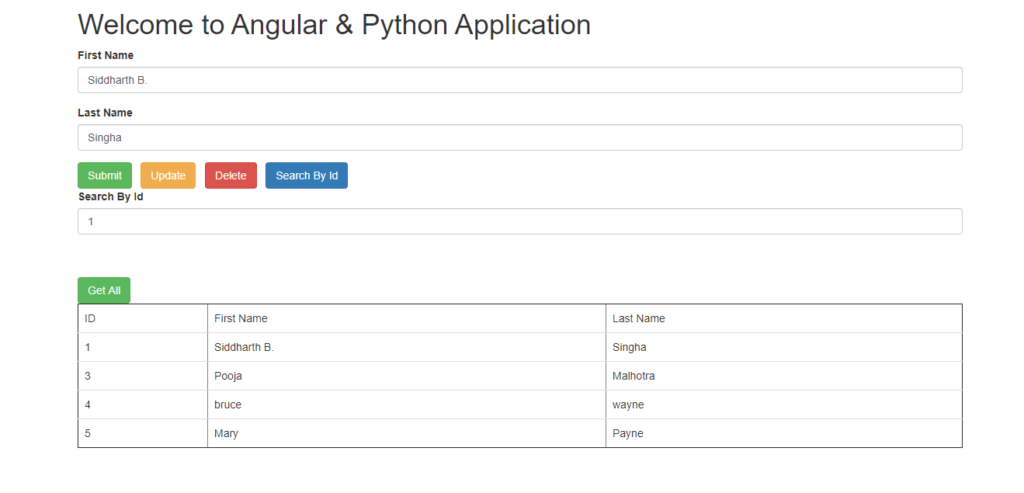
Thanks.
[…] http://datacyper.com/index.php/2020/04/02/angular-form/ […]