We will learn how to Build Application of your Favorite Cartoon Character in 20 Minutes.
In this article, we will randomly generate simpsons character images, names and their quotes
- Setting your Angular App
- Create a simple function and a click event
- Use axios module to make Http Request to an API End-point.
Step 1:
You should have node installed. Once done, just open your node terminal and type below commands
npm install -g @angular/cli
ng new my-first-app
cd simpson-app
ng serve
This should successfully start your server at port 4200
Step 2:
You should go into app.component.html and clean your whole introductory code, we will write our code in this file.
Add important Bootstrap cdn’s in index.html Or you could also add it through NPM or Yarn. As we are going to use bootstrap cards to display text and images
We are going to use an API end-point for simpsons.
This api randomly generates the name, quote and image of simpson character
https://thesimpsonsquoteapi.glitch.me/quotes
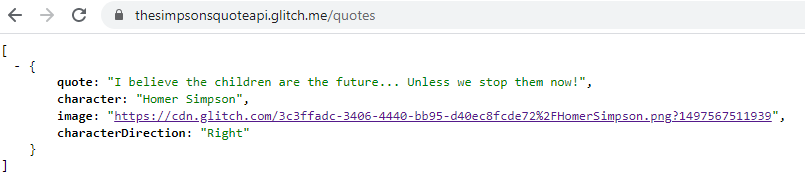
As you can see, this is a single array format and contains a single JSON response, we can fetch data from this API using axios Get request. Below is the full code to write in app.component.ts
Step 3:
import { Component, OnInit } from '@angular/core';
import axios from 'axios';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
title = 'my-quotes-app';
constructor() { }
simp_character: string;
simp_quotes: string;
simp_image: string;
ngOnInit() {
//alert("Hello Sidd")
this.someFunction();
}
someFunction() {
axios.get("https://thesimpsonsquoteapi.glitch.me/quotes")
.then((response)=> {
this.simp_character = response.data[0].character;
this.simp_quotes = response.data[0].quote;
this.simp_image = response.data[0].image;
})
.catch((error)=> {
console.log(error)
})
}
//https://thesimpsonsquoteapi.glitch.me/
clickEvent() {
this.someFunction();
}
}
Below is the code for app.component.html
<br >
<button (click)="clickEvent()" class="btn btn-success" style="margin-left: 300px; margin-right: 300px;">More from Simpsons</button>
<br >
<div class="card" style="width: 18rem;" style="margin-left: 300px; margin-right: 300px; background-color: aquamarine;">
<div class="card-body">
<h5 class="card-title">{{simp_character}}</h5>
<h6 class="card-subtitle mb-2 text-muted"></h6>
<p class="card-text">{{simp_quotes}}</p>
<img src = '{{simp_image}}'>
</div>
</div>
Go back to terminal type ng serve, and you should get outputs like these
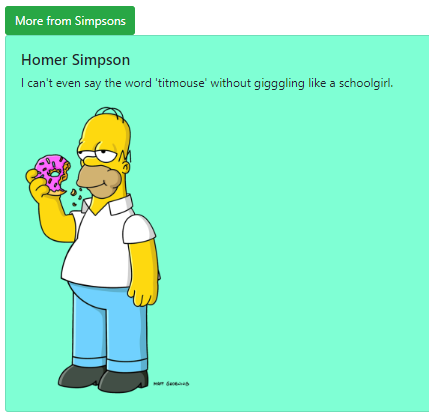
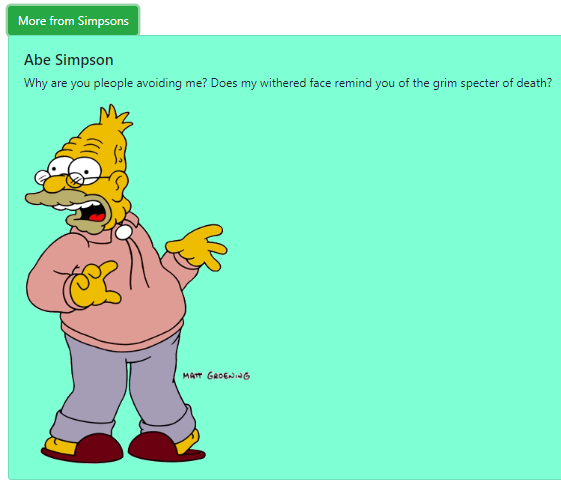
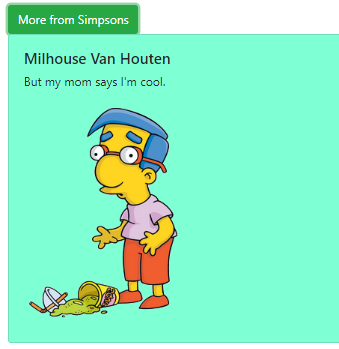
Thanks!!!
If you want Github repository for this code, please mention in the comment below.
I’m satisfied with the information that you provide for me and thanks for this because
sometimes people face this issue.
King regards,
Demir Duke
I am very much grateful for your effortsto putt on this article.
This article is updated, very informative and translucent.
Can I expect you will post this sort of another article in near future?
King regards,
Thompson Valenzuela
I’d say this is one of the best article I’ve read.
From beginning to end you nailed it. To write this you might have worked for research.
King regards,
Thomassen Dencker
Thanks for share this article it is quite helpful to me.
Best regards,
Balle Cannon
[…] developers opt for other languages over java as it works slow, compared to new programming […]