Generate QRcode and barcodes using Java
For maven project add this dependency in your POM file. Make sure version should not be less than 2.2 otherwise error will occurs in code.
<!-- https://mvnrepository.com/artifact/com.google.zxing/core -->
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>2.2</version>
</dependency>
For Java projects download zxing core 2.2 and then right click on project=>Build path=>configure build path=>Add External jars.
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.Hashtable;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
public class QRCode {
public static void main(String[] args) throws WriterException, IOException {
String qrCodeText = "Datacyper";//Write the QR code text
String filePath = "sample.png";// path where you want to save the file by default it saves in project folder
int size = 100;
String fileType = "png";//File extension name
File qrFile = new File(filePath);
generateQRCode(qrFile, qrCodeText, size, fileType);//pass the all parameters.
System.out.println("QRcode Generated!!");
}
public static void generateQRCode(File qrFile, String qrCodeText, int size, String fileType)
throws WriterException, IOException {
Hashtable<EncodeHintType, ErrorCorrectionLevel> hintMap = new Hashtable<>();
hintMap.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.L);
QRCodeWriter qrCodeWriter = new QRCodeWriter();
BitMatrix bitMatrix = qrCodeWriter.encode(qrCodeText, BarcodeFormat.QR_CODE, size, size, hintMap);
int matrixWidth = bitMatrix.getWidth();
BufferedImage image = new BufferedImage(matrixWidth, matrixWidth, BufferedImage.TYPE_INT_RGB);
image.createGraphics();
Graphics2D graphics = (Graphics2D) image.getGraphics();
graphics.setColor(Color.WHITE);
graphics.fillRect(0, 0, matrixWidth, matrixWidth);
graphics.setColor(Color.BLACK);
for (int i = 0; i < matrixWidth; i++) {
for (int j = 0; j < matrixWidth; j++) {
if (bitMatrix.get(i, j)) {
graphics.fillRect(i, j, 1, 1);
}
}
}
ImageIO.write(image, fileType, qrFile);
}
}
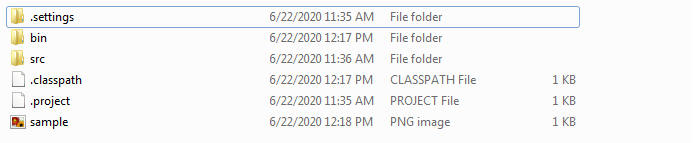
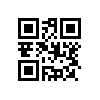
This is the generted QR code with text as Datacyper.
Thanks.