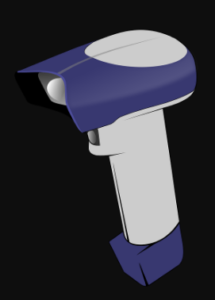
If you want to get any output from scanner device and wants to store data or manipulate the output data using java then follow these steps with Java Programming source code.
We use socket programming to achieve this. Socket programming is a way of connecting two nodes on a network to communicate with each other.
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.Socket;
import java.net.UnknownHostException;
/**
*
* @author Ravi kumar
* Datacyper
*
*/
public class ScannerSocket {
public static void main(String[] args) {
String ipAddress="192.142.6.51";//Ip address of the Scanner.
int port=2020;//port of the scanner
int timeOut=2000;//time out of the scanner
int qrRetryCount=10;//Number of times retry for scanning.
QRSCANDATA(ipAddress,port,timeOut,qrRetryCount);
}
public static void QRSCANDATA(String ipAddress, int port, int timeOut,int qrRetryCount)
{
Socket socket=null ;
InputStream is = null;
BufferedInputStream bis=null;
boolean flag = false;
try
{
socket = new Socket(ipAddress,port);
socket.setSoTimeout(timeOut);
int i=0;
while(i<qrRetryCount && !flag)
{
if(socket.isBound())
{
try {
is = socket.getInputStream();
bis=new BufferedInputStream(is);
String inputString = readInputStream(bis);
System.out.println("Scanned input------>"+inputString);
}
catch(UnknownHostException u)
{
System.out.println(u + ipAddress);
} finally {
bis.close();
is.close();
bis = null;
is = null;
}
}
i++;
Thread.sleep(500);
}
}
catch (Exception exception)
{
exception.printStackTrace();
} finally {
if(socket!=null){
try{
socket.close();
}catch(Exception e){}
socket=null;
}
}
}
private static String readInputStream(BufferedInputStream bis) throws IOException {
String data = "";
int s = bis.read();
if(s==-1)
return null;
data += ""+(char)s;
int len = bis.available();
if(len > 0) {
byte[] byteData = new byte[len];
bis.read(byteData);
data += new String(byteData);
}
return data;
}
}
This is a simple java code to get output from any scanner device.
In this i m passing 4 parameters to a QRSCANDATA function where in
1. first parameter i have passed the ip address of the device.
2.Second parameter is the port number of the device.
The client in socket programming must know two information: IP Address of Server and Port number.
3.Third parameter(Optional) is the timeout,it will try to scan the qrcode or brcode untill timeout.So you can set the time as per your requirement.
4.Fourth parameter(Optional) is the total try for scanning the QRcode or barcode.In this it will try 10 times to capture or scan the QRcode.I have use this because some device may takes more than one time to get the data from QRcode.
I hope this will help you in Java Programming. If you want any other clarification regarding this code then please feel free to contact us.
Thanks.
[…] the output signal can be supplied to the artificial neurons in the body, which will be received by the sensory […]