This is the simple Demo were we can see how to secure web application using spring boot.You can secure any URL or pages(HTML,JSP etc) by giving permit to its pages.
Spring Security is highly customizable authentication and access control framework. It focus on both authentication and authorization to java applications.It is the de-facto standard for securing Spring based applications.
Before Starting project be ensure you have already installed spring suit tools in Eclipse IDE or using spring suit tools.
How to Secure Web Application using Spring Boot.
1.Create a new Web project by File >> New >> Spring Starter Project.
2.Give the name of the project as “WebSecure” and then click Next.
3.Select “Spring Security”,”Spring Web”,”Spring Web Services” and then 4.click finish.
This is the project structure.
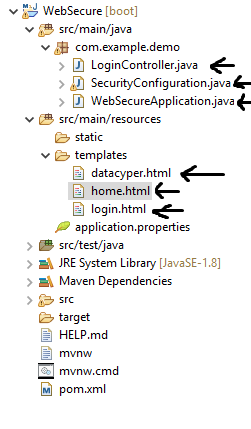
Create 5 file as shown below.WebSecureApplication.java will automatically created with the project.
login.html
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="https://www.thymeleaf.org"
xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3">
<head>
<meta charset="ISO-8859-1">
<title>Login</title>
</head>
<body>
<div th:if="${param.error}">
<h3>The username or password is incorrect!!</h3>
</div>
<div th:if="${param.logout}">
<h3>The user has been logged out</h3>
</div>
<form th:action="@{/login}" method="post">
<div><label>User Name :<input type text="text" name="username"></label></div>
<div><label>Password : <input type="password" name="password"></label></div>
<div><input type="submit" value="login"></div>
</form>
</body>
</html>
home.html
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Secure Web Application</title>
</head>
<body>
<h1>Welcome to Datacyper</h1>
<h3><a th:href="@{/datacyper}">LOGIN</a></h3>
</body>
</html>
datacyper.html
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="https://www.thymeleaf.org"
xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3">
<head>
<meta charset="ISO-8859-1">
<title> Welcome to Datacyper</title>
</head>
<body>
<h1 th:incline="text">Hi [[${#httpServletRequest.remoteUser}]]!</h1>
<p>Welcome to Datacyper</p>
<form th:action="@{/logout}" method="post">
<input type="submit" value="Log Out"/>
</form>
</body>
</html>
LoginController.java
package com.example.demo;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ViewControllerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class LoginController implements WebMvcConfigurer {
public void addviewControllers(ViewControllerRegistry registry) {
//This will map your URl with your html files. registry.addViewController("/home").setViewName("views/home");
registry.addViewController("/").setViewName("home");
registry.addViewController("/datacyper").setViewName("datacyper");
registry.addViewController("/login").setViewName("login");
}
}
SecurityConfiguration.java
package com.example.demo;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.provisioning.InMemoryUserDetailsManager;
@Configuration
@EnableWebSecurity
public class SecurityConfiguration extends WebSecurityConfigurerAdapter{
@Override
protected void configure(HttpSecurity http) throws Exception{
http.authorizeRequests()
.antMatchers("/","/home").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Bean
@Override
public UserDetailsService userDetailsService()
{
UserDetails user= User.withDefaultPasswordEncoder().username("datacyper")
.password("pass123")
.roles("USER")
.build();
return new InMemoryUserDetailsManager(user);
}
}
If you want to change port then go to application.properties file and write : server.port=8081
If you have any queries than feel free to contact us or give comment below.
Thanks.